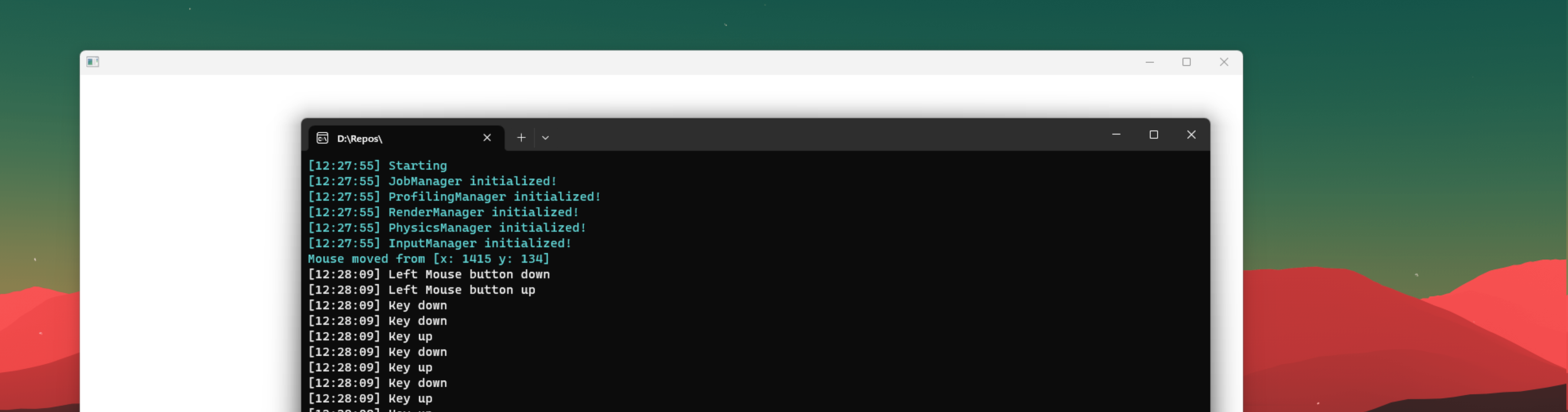
PROJEKT CCE
Project CCE is a private project, in which I can apply many theoretical concepts in practice.
The focus is on implementing some engine systems in C++. I started this project in January
2023 and since then the project has developed a lot.
Current features include among others:
- Strings
- Memory Allocation
- Basic D3D11 Rendering
- Job System
- Logging
- Runtime Debugging Tools (ImGui)
- Input (Maus & Tastatur, XInput)
STRINGS
Strings are an elementary component of all software.
They play a particularly critical role in games
since their immutability makes
them a performance-critical factor in common operations.
Inspired by Jason Gregory's Game Engine Architecture,
I have implemented my own string class that optimizes some operations.
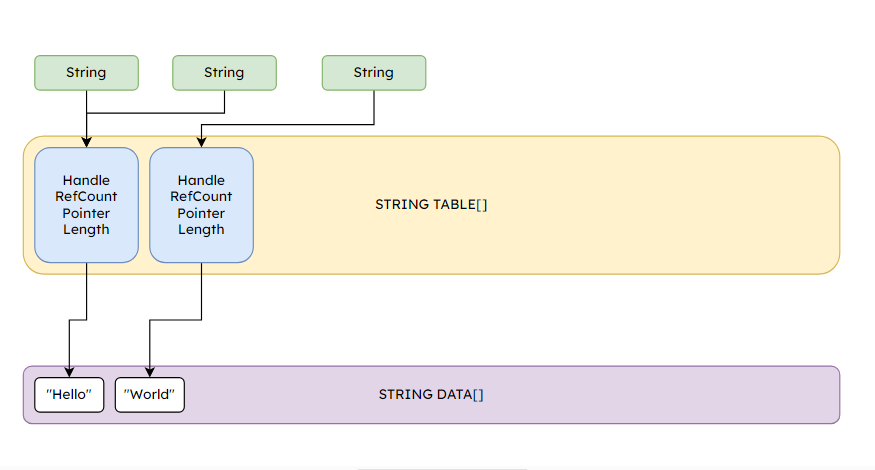
The performance of various operations is listed below.
All tests were averaged over 1,000,000 operations in the release configuration.
Operation | CCE::String | std::string |
---|---|---|
Create New | 137 ns | 199 ns |
Create Existing | 68 ns | 328 ns |
Copy | 136 ns | 58 ns |
Move | 129 ns | 23 ns |
Length | 26 ns | 23 ns |
Compare | 26 ns | 79 ns |
Append | 395 ns | 65 ns |
Destroy | 1898 ns | 304 ns |
Destruction of the strings is currently still very time-consuming, as defragmentation is always carried out during this process.
This operation can later be carried out over several frames and/or not with every destruction, but only if necessary.
The overhead when appending strings can also be clearly seen. The data array is always traversed to find the appropriate strings.
Also noticeable is the pointer indirection, which I would like to optimize in the future.
This implementation offers optimization in some operations, but is not fundamentally "better" than other models.
Either way, it is an exciting challenge that has introduced me to the implementation details of some basic optimization concepts
such as a cache-friendly data layout and hashing.